--fail-fast and learn fast using PRY!
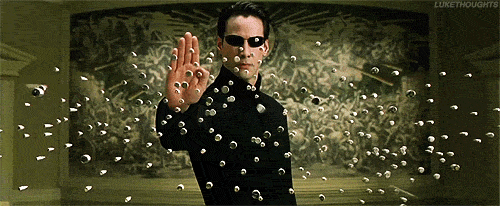
Today we are going to be talking about a very useful ruby developer tool called Pry.
What is Pry, what does it do?
From the official pry website
Pry is a powerful alternative to the standard IRB shell for Ruby. It features syntax highlighting, a flexible plugin architecture, runtime invocation and source and documentation browsing.
What this basically means for our beginner minds is that we can insert a little snippet of code to something we want to debug or take a closer look at.
We can essentially freeze time and arrange bullets just like Neo from the Matrix
Here is a basic example using arrays:
one_piece_characters = ["luffy", "zoro", "nami", "usopp", "sanji", "chopper", "robin", "franky", "brook"]
# I don't know at what index "chopper" is
# I also want to "puts" the names of the characters once in the screen
Pry to the rescue!
simply put this little code
require 'pry'
one_piece_characters = ["luffy", "zoro", "nami", "usopp", "sanji", "chopper", "robin", "franky", "brook"]
binding.pry
This will freeze your code at this instance and you will be able to run an IRB (Interactive Ruby Shell).
It will look something like this:
1: require 'pry'
2:
3: one_piece_characters = ["luffy", "zoro", "nami", "usopp", "sanji", "chopper", "robin", "franky", "brook"]
4:
5:
6: binding.pry
[1] pry(main)>
From here, you'll be able to examine the array of one_piece_characters
and its values.
[1] pry(main)> one_piece_characters[0]
=> "luffy"
[2] pry(main)> one_piece_characters[5]
=> "chopper"
I see, so "chopper" is at index 5 in the array. Now I want to "puts" each
of the characters' names in the array. How the heck do I do that!?
Well, this is what I know so far: one_piece_characters
is an array, and arrays have different methods in which we can call on them.
The method we are looking for is .each
, this method allows us to do something to each object in the array.
Let's play with the .each
method with our array!
1: require 'pry'
2:
3: one_piece_characters = ["luffy", "zoro", "nami", "usopp", "sanji", "chopper", "robin", "franky", "brook"]
4:
5: one_piece_characters.each do |display_name|
6: binding.pry
7: end
[1] pry(main)> "#{display_name}"
=> "luffy"
[2] pry(main)> "#{display_name}"
=> "luffy"
What's going on? Why isn't the second item in the array not showing up?
-
We are inside a loop at one instance, this is why the name "luffy" is still showing up. We have to
exit
this loop first in order to see the next instance of the loop. Try it out~4: ...
5: one_piece_characters.each do |display_name|
6: binding.pry
7: end[1] pry(main)> "#{display_name}"
=> "luffy"
[2] pry(main)> "#{display_name}"
=> "luffy"
[3] pry(main)> exit
Now we see the same loop again:
4: ...
5: one_piece_characters.each do |display_name|
6: binding.pry
7: end
[1] pry(main)> "#{display_name}"
=> "zoro"
[2] pry(main)> "#{display_name}"
=> "zoro"
But this time is different because the loop is running and the next instance is at index 1, when we type exit
again, it will be at index 2, etc, etc, until the .each
method finishes iterating the whole array.
TIP: if you don't want to see the whole iteration, you can simply type exit!
and this will force quit pry.
Now that we played with pry and know how the .each
method works, we can type this:
5: one_piece_characters.each do |display_name|
6: puts "Hello my name is #{display_name}!"
7: end
It will display this:
Hello my name is luffy!
Hello my name is zoro!
Hello my name is nami!
Hello my name is usopp!
Hello my name is sanji!
Hello my name is chopper!
Hello my name is robin!
Hello my name is franky!
Hello my name is brook!
AWESOME!!!! NOW I WANNA TRYYYYYYY! -(๑☆‿ ☆#)ᕗ